LitPop class¶
This class is a data model of economic exposure. It models countries’ gridded exposure by interpolating an macroeconomic indicator through the product of night light intensities and gridded population count for a specific year. Asset value is distributed to the grid proportzional to LitPop. LitPop at each pixel is calculated in the script as:
\(Lit^mPop^n = Lit^m * Pop^n\), with \(exponents = [m, n] \in \N_0\) (Default values are \(m=n=1\)).
The night light images used are the following: - year 2012 and 2016: https://earthobservatory.nasa.gov/Features/NightLights (15 arcsec resolution (~500m))
The gridded population count data used are the following: - Gridded Population of the World (GPW), v4: Population Count, v4.10, v4.11 or later versions (2000, 2005, 2010, 2015, 2020) http://sedac.ciesin.columbia.edu/data/collection/gpw-v4/sets/browse (i.e. http://sedac.ciesin.org/data/set/gpw-v4-population-count-rev10 or http://sedac.ciesin.org/data/set/gpw-v4-population-count-rev11 etc.)
Always the GPW file of the year closest to the target year is required. To download GPW data you need to register on the SEDAC website (for free). Then you can access the download site, i.e.: http://sedac.ciesin.columbia.edu/data/set/gpw-v4-population-count-rev11/data-download. There, choose the settings as shown, ticking the box of the years you need: The population data from GWP needs to be downloaded manually as TIFF from this site and placed in
the system data folder of climada, i.e.: climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif.
Direct download links are avilable, also for older versions, i.e.: http://sedac.ciesin.columbia.edu/downloads/data/gpw-v4/gpw-v4-population-count-rev10/gpw-v4-population-count-rev10_2015_30_sec_tif.zip, or: http://sedac.ciesin.columbia.edu/downloads/data/gpw-v4/gpw-v4-population-count-rev11/gpw-v4-population-count-rev11_2015_30_sec_tif.zip
All other required data is acquired automatically.
Available macroeconomic indicators can be selected via the variable fin_mode: - ‘pc’: produced capital (Source: World Bank DataBank), incl. manufactured or built assets such as machinery, equipment, and physical structures (pc is in constant 2014 USD, data is available for the years 1995, 2000, 2005, 2010, 2014). The pc-data is stored in the subfolder data/system/Wealth-Accounts_CSV/. Source: https://datacatalog.worldbank.org/dataset/wealth-accounting - ‘gdp’: gross-domestic product (Source: World Bank) - ‘income_group’: gdp multiplied by country’s income group+1 - ‘nfw’: households’ non-financial wealth (Source: Credit Suisse, of households only) - ‘tw’: households’ total wealth (Source: Credit Suisse, of households only)
Regarding the GDP (nominal GDP at current USD) and income group values, they are obtained from the World Bank using the pandas-datareader API. If a value is missing, the value of the closest year is considered. When no values are provided from the World Bank, we use the Natural Earth repository values.
Initiating LitPop():
The LitPop
class inherits from the `Exposures
<climada_entity_Exposures.ipynb#Exposures-class>`__ class. It provides a set_country()
method which enables to compute exposure data for a country using different settings and input data. The first time a nightlight image is used, it is downloaded and stored locally. This might take some time.
NOTE / Downloading existing LitPop exposure data:
Readily computed LitPop exposure data, distributing produced capital of 2014 at a resolution of 30 arcsec can be downloaded from the ETH Research Repository: https://doi.org/10.3929/ethz-b-000331316. The dataset contains gridded data for more than 200 countries as CSV files. [These can be imported into Python using the Exposures class, i.e. Exposures(pd.read_csv(file_name))]
[1]:
# Import required packages:
import numpy as np
import pandas as pd
from matplotlib import colors
from iso3166 import countries as iso_cntry
from climada.entity.exposures.litpop import LitPop
2019-05-15 07:58:21,133 - climada - DEBUG - Loading default config file: /Users/aznarsig/Documents/Python/climada_python/climada/conf/defaults.conf
Country Exposure¶
In the following, we will create exposure data sets and plots for a variety of countries, comparing different settings. ### Default Settings
[2]:
# Initiate a default LitPop exposure entity for Switzerland (ISO3-Code 'CHE'):
ent = LitPop()
ent.set_country('CHE')
""" In case you encounter an error here, because the GPW data is missing: The gridded population count data used are the following:
- Gridded Population of the World (GPW), v4: Population Count, v4.10 or later (2000, 2005, 2010, 2015, 2020).
http://sedac.ciesin.columbia.edu/data/collection/gpw-v4/sets/browse
The population data from GWP needs to be downloaded manually as TIFF
from this site and placed in the system data folder of climada,
i.e.: climada_python/data/system/gpw_v4_population_count_rev10_2015_30_sec.tif
"""
2019-05-15 07:58:41,885 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 30.0 arcsec.
2019-05-15 07:58:41,886 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 07:58:41,887 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_C1_geo_gray.tif.
2019-05-15 07:58:56,891 - climada.entity.exposures.gpw_import - INFO - Reference year: 2016. Using nearest available year for GWP population data: 2015
2019-05-15 07:58:56,892 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 07:58:56,892 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 07:59:12,739 - climada.util.finance - INFO - GDP CHE 2014: 7.092e+11.
2019-05-15 07:59:13,653 - climada.util.finance - INFO - GDP CHE 2016: 6.702e+11.
2019-05-15 07:59:14,150 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 07:59:14,157 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 07:59:14,715 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 51 s
2019-05-15 07:59:14,716 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 07:59:14,716 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 07:59:14,717 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 07:59:14,717 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 07:59:14,719 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 07:59:14,721 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 07:59:14,722 - climada.entity.exposures.base - INFO - geometry not set.
[2]:
' In case you encounter an error here, because the GPW data is missing: The gridded population count data used are the following:\n- Gridded Population of the World (GPW), v4: Population Count, v4.10 or later (2000, 2005, 2010, 2015, 2020).\nhttp://sedac.ciesin.columbia.edu/data/collection/gpw-v4/sets/browse\nThe population data from GWP needs to be downloaded manually as TIFF\nfrom this site and placed in the system data folder of climada,\ni.e.: climada_python/data/system/gpw_v4_population_count_rev10_2015_30_sec.tif\n'
Plotting¶
The exposure entity was initiated using the default setting, i.e. a resolution of 30 arcsec and produced capital ‘pc’ as total asset value, using the exponents \([1, 1]\).
If the init worked, we can now plot a map of Switzerland’s exposure. We can get a good impression of the exposure distribution in Switzerland by comparing plots with linear and log-normal colormaps:
(please refer to the exposure tutorial climada_entity_Exposures.ipynb for more info on the visualization of exposure data.)
[3]:
ent.set_geometry_points()
# plot exposure with linear colormap and major towns (pop_name=True):
ent.plot_hexbin(pop_name=True)
# plot exposure with log-normal colormap:
norm=colors.LogNorm(vmin=500, vmax=4.0e9)
ent.plot_hexbin(norm=norm)
2019-05-15 07:59:14,778 - climada.entity.exposures.base - INFO - Setting geometry attribute.
/Users/aznarsig/anaconda3/envs/climada_up/lib/python3.7/site-packages/matplotlib/tight_layout.py:176: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all axes decorations.
warnings.warn('Tight layout not applied. The left and right margins '
[3]:
(<Figure size 648x936 with 2 Axes>,
array([[<cartopy.mpl.geoaxes.GeoAxesSubplot object at 0x1a2fa0a2b0>]],
dtype=object))
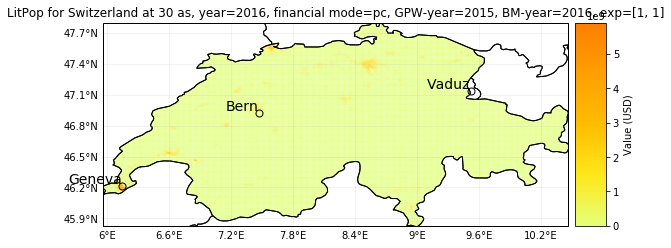
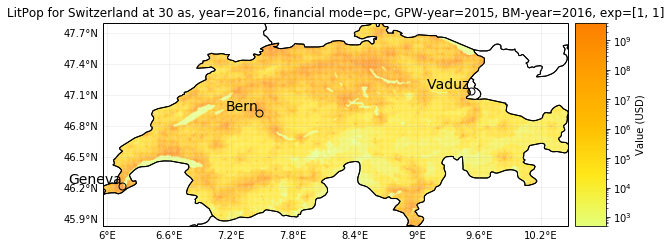
Settings: fin_mode¶
Instead on produced capital, we can also downscale other available macroeconomic indicators as estimates of asset value. The indicator can be set via the variable fin_mode:
'pc': produced capital (Source: World Bank DataBank), incl. manufactured or built assets such as machinery, equipment, and physical structures (pc is in constant 2014-USD, data available for the years 1995, 2000, 2005, 2010, 2014)
'gdp': gross-domestic product (Source: World Bank)
'income_group': gdp multiplied by country's income group+1
'nfw': households' non-financial wealth (Source: Credit Suisse, of households only)
'tw': households' total wealth (Source: Credit Suisse, of households only)
Let’s initiate the entity for Switzerland with the financial mode “income_group”:
[4]:
# Initiate a default LitPop exposure entity for Switzerland (ISO3-Code 'CHE'):
ent = LitPop()
ent.set_country('CHE', fin_mode='income_group') # try other fin_modes: 'gdp', 'nfw', 'pc'
# plot exposure:
ent.plot_hexbin(pop_name=True)
2019-05-15 07:59:45,714 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 30.0 arcsec.
2019-05-15 07:59:45,715 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 07:59:45,716 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_C1_geo_gray.tif.
2019-05-15 08:00:00,521 - climada.entity.exposures.gpw_import - INFO - Reference year: 2016. Using nearest available year for GWP population data: 2015
2019-05-15 08:00:00,522 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:00:00,523 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:00:12,950 - climada.util.finance - INFO - GDP CHE 2016: 6.702e+11.
2019-05-15 08:00:13,013 - climada.util.finance - INFO - Income group CHE 2016: 4.
2019-05-15 08:00:13,434 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:00:13,442 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:00:14,036 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 45 s
2019-05-15 08:00:14,037 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:00:14,038 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:00:14,039 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:00:14,039 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:00:14,040 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:00:14,040 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:00:14,041 - climada.entity.exposures.base - INFO - geometry not set.
/Users/aznarsig/anaconda3/envs/climada_up/lib/python3.7/site-packages/matplotlib/tight_layout.py:176: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all axes decorations.
warnings.warn('Tight layout not applied. The left and right margins '
[4]:
(<Figure size 648x936 with 2 Axes>,
array([[<cartopy.mpl.geoaxes.GeoAxesSubplot object at 0x1a33520b70>]],
dtype=object))
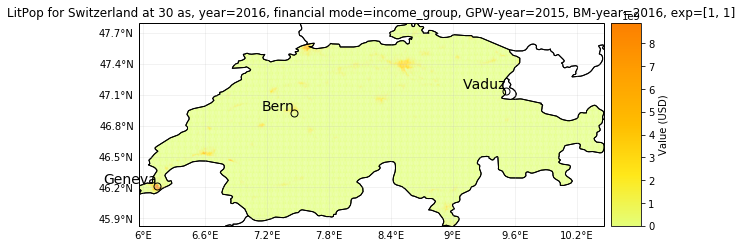
This map shows like the default one above, the only difference is the absolute value per pixel: Note that the range of the color scale has changed because the total asset value estimated with the income_group mode is larger than produced capital stock.
Settings: resolution (res_arcsec) and reference_year¶
We can also change the resolution in arc seconds to one of these values: 30, 60, 120, 300, 600, 3600.
\(30 arcsec \approx 1 km\).
\(3600 arcsec = 1\degree \approx 110 km\).
Additionally, we can change the year our exposure is supposed to represent. For this, nightlight and population data are used that are closest to the requested years. Macroeconomic indicators like produced capital are interpolated from available data or scaled proportional to GDP.
Let’s estimate a produced capital exposure map for Switzerland in 2017 with a resolution of 300 arcsec:
[5]:
ent = LitPop()
ent.set_country('CHE', fin_mode='pc', res_arcsec=300, reference_year=2017)
ent.set_geometry_points()
# plot exposure with larger line width for better visibility:
ent.plot_hexbin(linewidth=7)
# Instead of linewight, the gridsize can be adjusted for better visibility (low values --> big markers).
# Warning: If gridsize is changed, the plot doesn't reflect the actual data's grid anymore,
# (please refer to the exposure tutorial *climada_entity_Exposures.ipynb*
# for more info on the visualization of exposure data.)
ent.plot_hexbin(gridsize=20)
2019-05-15 08:00:39,281 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 300 arcsec.
2019-05-15 08:00:39,282 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 08:00:39,282 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_C1_geo_gray.tif.
2019-05-15 08:00:42,604 - climada.entity.exposures.gpw_import - INFO - Reference year: 2017. Using nearest available year for GWP population data: 2015
2019-05-15 08:00:42,605 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:00:42,605 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:00:55,337 - climada.util.finance - INFO - GDP CHE 2014: 7.092e+11.
2019-05-15 08:00:55,873 - climada.util.finance - INFO - GDP CHE 2017: 6.790e+11.
2019-05-15 08:00:55,980 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:00:55,981 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:00:55,994 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 34 s
2019-05-15 08:00:55,995 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:00:55,996 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:00:55,997 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:00:55,998 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:00:55,998 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:00:55,999 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:00:56,000 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:00:56,010 - climada.entity.exposures.base - INFO - Setting geometry attribute.
/Users/aznarsig/anaconda3/envs/climada_up/lib/python3.7/site-packages/matplotlib/tight_layout.py:176: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all axes decorations.
warnings.warn('Tight layout not applied. The left and right margins '
[5]:
(<Figure size 648x936 with 2 Axes>,
array([[<cartopy.mpl.geoaxes.GeoAxesSubplot object at 0x1a275826a0>]],
dtype=object))
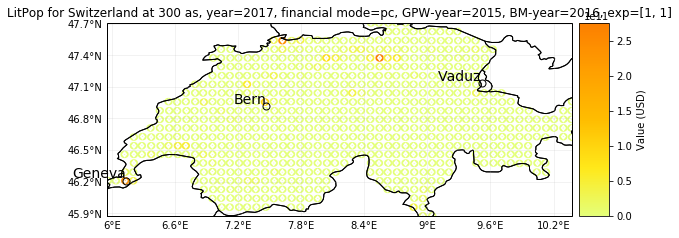
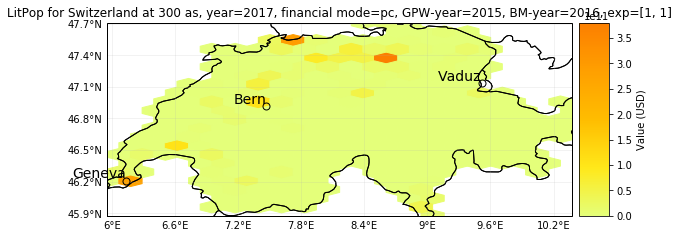
As can you can read from the logger output above the plot, the following data time steps were used to estimate 2017’s exposure: - GPW Population of 2015 (closest year) - BlackMarble Nightlights of 2016 (closest year) - Produced capital of 2014 (closest year), inflated proportional to the GDP increase from 2014 to 2017. This is an inflation of roughly 4.5% in this case.
Settings: exponents of \(Lit^mPop^n\)¶
LitPop per pixel is the product of the exponents of nightlights+1 (Lit) and Population count (Pop).
\(Lit^mPop^n = Lit^m * Pop^n\), with \(exponents = [m, n] \in \N_0\) (Default values are \(m=n=1\)).
We can also change m and n.
For countries with badly resolved population data (i.e. Jamaica or Uzbekistan), it can make sense to use nightlights only, i.e. an high exponent of Lit instead of LitPop. Note that if Pop is not used, \(Lit = nightlights\) instead of \(nightlights+1\).
Let’s compare exposure maps for \(LitPop=Lit^1Pop^1\) (default); \(Lit^3\); and \(Pop^1\) Jamaica at a resolution of 60 arcsec.
Please execute one cell after the other:
[6]:
ent = LitPop()
res = 60
country = 'JAM' # Try different countries, i.e. 'JAM', 'CHE', 'RWA', 'MEX'
markersize = 3
gridsize = 70
buffer_deg=.04
# The arguments 'exponent' is used to set the power with which Lit and Pop go into LitPop:
ent.set_country(country, res_arcsec=res, reference_year=2014, exponents=[0, 1]) # Population only
ent.set_geometry_points()
ent.plot_hexbin(gridsize = gridsize, linewidth=markersize, buffer=buffer_deg)
# note: buffer_deg adds a buffer around the plotted data to show the whole country
"""Lit^0 * Pop^1"""
2019-05-15 08:01:08,283 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 60 arcsec.
2019-05-15 08:01:08,284 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 08:01:08,285 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_B1_geo_gray.tif.
2019-05-15 08:01:15,359 - climada.entity.exposures.gpw_import - INFO - Reference year: 2014. Using nearest available year for GWP population data: 2015
2019-05-15 08:01:15,360 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:01:15,361 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:01:34,125 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:01:34,126 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:01:34,157 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 36 s
2019-05-15 08:01:34,158 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:01:34,158 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:01:34,159 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:01:34,159 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:01:34,160 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:01:34,160 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:01:34,161 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:01:34,172 - climada.entity.exposures.base - INFO - Setting geometry attribute.
[6]:
'Lit^0 * Pop^1'
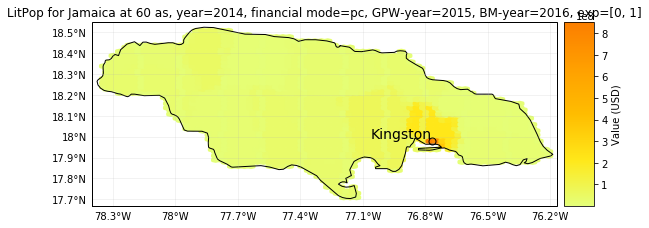
[7]:
# JAMAICA:
ent.set_country(country, res_arcsec=res, reference_year=2014, exponents=[3, 0]) # Nightlights^3 only
ent.set_geometry_points()
ent.plot_hexbin(gridsize = gridsize, linewidth=markersize, buffer=buffer_deg)
"""Lit^3"""
2019-05-15 08:01:44,827 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 60 arcsec.
2019-05-15 08:01:44,828 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 08:01:44,828 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_B1_geo_gray.tif.
2019-05-15 08:01:51,921 - climada.entity.exposures.gpw_import - INFO - Reference year: 2014. Using nearest available year for GWP population data: 2015
2019-05-15 08:01:51,922 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:01:51,923 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:02:09,945 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:02:09,946 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:02:09,982 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 34 s
2019-05-15 08:02:09,983 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:02:09,983 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:02:09,984 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:02:09,985 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:02:09,986 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:02:09,986 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:02:09,987 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:02:09,998 - climada.entity.exposures.base - INFO - Setting geometry attribute.
[7]:
'Lit^3'
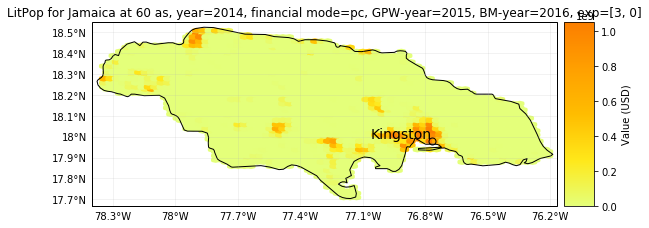
[8]:
ent.set_country(country, res_arcsec=res, reference_year=2014, exponents=[1, 1]) # Default LitPop [1, 1]
ent.set_geometry_points()
ent.plot_hexbin(gridsize = gridsize, linewidth=markersize, buffer=buffer_deg)
"""Lit^1 * Pop^1"""
2019-05-15 08:02:21,246 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 60 arcsec.
2019-05-15 08:02:21,247 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 08:02:21,248 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_B1_geo_gray.tif.
2019-05-15 08:02:28,388 - climada.entity.exposures.gpw_import - INFO - Reference year: 2014. Using nearest available year for GWP population data: 2015
2019-05-15 08:02:28,389 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:02:28,389 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:02:46,815 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:02:46,816 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:02:46,866 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 35 s
2019-05-15 08:02:46,867 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:02:46,868 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:02:46,869 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:02:46,871 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:02:46,873 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:02:46,876 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:02:46,878 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:02:46,898 - climada.entity.exposures.base - INFO - Setting geometry attribute.
[8]:
'Lit^1 * Pop^1'
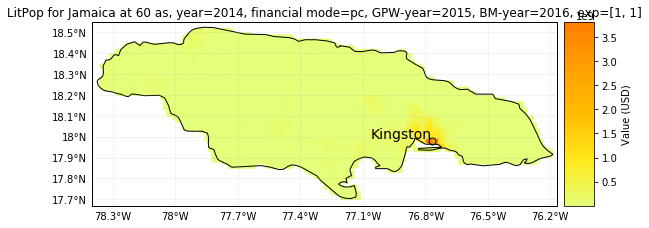
For Switzerland, population is resolved on the 3rd administrative level, with 2538 distinct geographical units. Therefore, the purely population-based map is highly resolved.
For Jamaica, population is only resolved on the 1st administrative level, with only 14 distinct geographical units. Therefore, the purely population-based map shows large monotonous patches. Still, the combination of Lit and Pop results in a concentration of asset value estimates around the capital city Kingston.
Multiple countries¶
Computing LitPop-entities for a list of countries and plot a log-normal exposure map:
[9]:
# Initiate GDP-Entity for Tanzania, Rwanda, and Burundi:
ent = LitPop()
countries_list = ['BDI', 'RWA', 'TZA']
ent.set_country(countries_list, res_arcsec=120, reference_year=2014, fin_mode='gdp')
ent.set_geometry_points()
2019-05-15 08:03:24,391 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 120 arcsec.
2019-05-15 08:03:24,393 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 08:03:24,394 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_C2_geo_gray.tif.
2019-05-15 08:03:28,799 - climada.entity.exposures.gpw_import - INFO - Reference year: 2014. Using nearest available year for GWP population data: 2015
2019-05-15 08:03:28,800 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:03:28,801 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:03:43,133 - climada.util.finance - INFO - GDP TZA 2014: 4.996e+10.
2019-05-15 08:03:44,342 - climada.util.finance - INFO - GDP RWA 2014: 8.017e+09.
2019-05-15 08:03:45,605 - climada.util.finance - INFO - GDP BDI 2014: 2.706e+09.
2019-05-15 08:03:45,721 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:03:45,722 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:03:45,970 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:03:45,971 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:03:46,127 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 7
2019-05-15 08:03:46,128 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 0
2019-05-15 08:03:46,542 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:03:46,550 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:03:47,094 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 58 s
2019-05-15 08:03:47,095 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:03:47,095 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:03:47,095 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:03:47,096 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:03:47,097 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:03:47,098 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:03:47,098 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:03:47,135 - climada.entity.exposures.base - INFO - Setting geometry attribute.
[10]:
# Plot GDP-Entity for Tanzania, Rwanda, and Burundi:
# ent.plot_hexbin(linewidth=2, buffer=.3) # plot using linear colormap
# ent.plot_hexbin(linewidth=2, buffer=.3, bins='log') # plot using logarithmic colormap
norm=colors.LogNorm(vmin=1.5e2, vmax=1.5e9) # setting range for the log-normal scale
ent.plot_hexbin(linewidth=2, buffer=.3, norm=norm) # log-normal colormap from USD 150 to USD 1'500'000'000.
"""GDP downscaling with LitPop for Tanzania, Rwanda, and Burundi (2014)"""
/Users/aznarsig/anaconda3/envs/climada_up/lib/python3.7/site-packages/matplotlib/tight_layout.py:176: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all axes decorations.
warnings.warn('Tight layout not applied. The left and right margins '
[10]:
'GDP downscaling with LitPop for Tanzania, Rwanda, and Burundi (2014)'
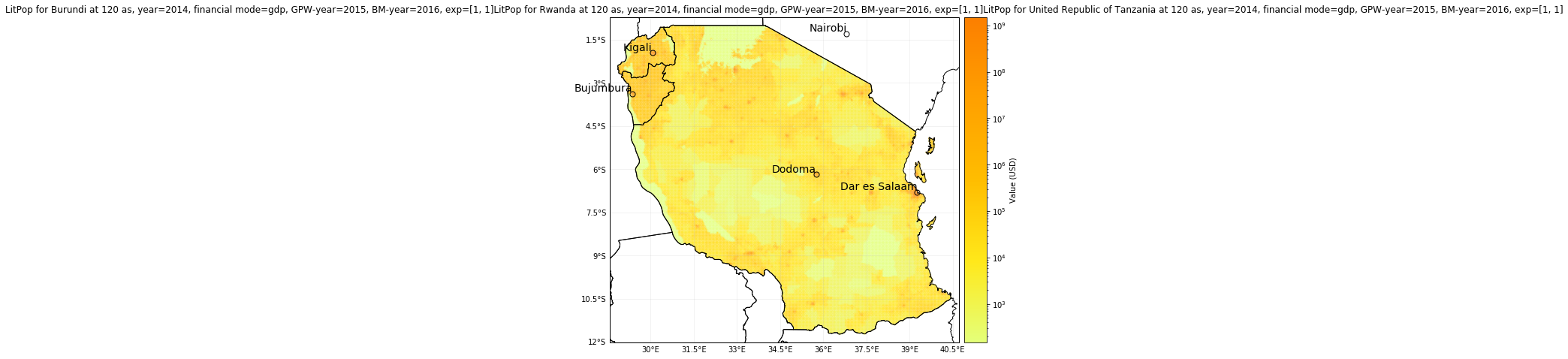
Sub-national (admin-1) GDP as intermediate downscaling layer¶
In order to improve downscaling for countries with large regional differences within, a subnational breakdown of GDP can be used as an intermediate downscaling layer wherever available.
The sub-national (admin-1) GDP-breakdown needs to be added manually as a “.xls”-file to the folder data/system/GSDP/ in the CLIMADA-directory. Currently, such data is provided for more than 10 countries, including USA, India, and China.
The xls-file requires at least the following columns (with names specified in row 1): - State_Province: Names of admin-1 regions, i.e. states, cantons, provinces. Names need to match the naming of admin-1 shapes in the data used by the python package cartopy.io (c.f. shapereader.natural_earth(name=’admin_1_states_provinces’)) - GSDP_ref: value of sub-national GDP to be used (absolute or relative values) - Postal (optional): Alternative identifier of region, if names do not match wioth cartopy. Needs to correspond to the Postal-identifiers used in the shapereader of cartopy.io.
Please note that while admin1-GDP will per definition improve the downscaling of GDP, it might not neccessarily improve the downscaling quality for other asset bases like produced capital (pc).
How To:
The intermediadte downscaling layer can be activated with the option admin1_calc.
The option adm1_scatter produces a scatter plot to compare the modelled and observed GDP per admin-1 region.
[11]:
# Initiate GDP-Entity for Switzerland, with and without admin1_calc:
ent_adm0 = LitPop()
ent_adm0.set_country('CHE', res_arcsec=30, fin_mode='gdp', admin1_calc=False)
ent_adm0.set_geometry_points()
ent_adm1 = LitPop()
ent_adm1.set_country('CHE', res_arcsec=30, fin_mode='gdp', admin1_calc=True)
ent_adm0.check()
ent_adm0.set_geometry_points()
ent_adm1.check()
ent_adm1.set_geometry_points()
print('Done.')
2019-05-15 08:04:11,491 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 30 arcsec.
2019-05-15 08:04:11,492 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 08:04:11,492 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_C1_geo_gray.tif.
2019-05-15 08:04:26,478 - climada.entity.exposures.gpw_import - INFO - Reference year: 2016. Using nearest available year for GWP population data: 2015
2019-05-15 08:04:26,479 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:04:26,479 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:04:38,972 - climada.util.finance - INFO - GDP CHE 2016: 6.702e+11.
2019-05-15 08:04:39,403 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:04:39,409 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:04:39,975 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 45 s
2019-05-15 08:04:39,975 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:04:39,976 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:04:39,977 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:04:39,977 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:04:39,978 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:04:39,979 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:04:39,980 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:04:40,019 - climada.entity.exposures.base - INFO - Setting geometry attribute.
2019-05-15 08:04:57,914 - climada.entity.exposures.litpop - INFO - Generating LitPop data at a resolution of 30 arcsec.
2019-05-15 08:04:57,915 - climada.entity.exposures.nightlight - DEBUG - Found all required satellite data (1 files) in folder /Users/aznarsig/Documents/Python/climada_python/data/system
2019-05-15 08:04:57,917 - climada.entity.exposures.litpop - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/BlackMarble_2016_C1_geo_gray.tif.
2019-05-15 08:05:12,532 - climada.entity.exposures.gpw_import - INFO - Reference year: 2016. Using nearest available year for GWP population data: 2015
2019-05-15 08:05:12,533 - climada.entity.exposures.gpw_import - INFO - GPW Version v4.11
2019-05-15 08:05:12,533 - climada.entity.exposures.gpw_import - DEBUG - Importing /Users/aznarsig/Documents/Python/climada_python/data/system/gpw_v4_population_count_rev11_2015_30_sec.tif
2019-05-15 08:05:40,098 - climada.util.finance - INFO - GDP CHE 2016: 6.702e+11.
2019-05-15 08:05:40,519 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:40,527 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:41,114 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Valais.
2019-05-15 08:05:41,144 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:41,146 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:41,667 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Ticino.
2019-05-15 08:05:41,681 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:41,682 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:42,089 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Graubünden.
2019-05-15 08:05:42,131 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:42,133 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:42,752 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Schaffhausen.
2019-05-15 08:05:42,753 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 2
2019-05-15 08:05:42,754 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 0
2019-05-15 08:05:42,758 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:42,759 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:43,033 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Thurgau.
2019-05-15 08:05:43,039 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:43,040 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:43,347 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Zürich.
2019-05-15 08:05:43,355 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:43,356 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:43,704 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Aargau.
2019-05-15 08:05:43,713 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:43,714 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:44,041 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Basel-Stadt.
2019-05-15 08:05:44,042 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:44,043 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:44,295 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Basel-Landschaft.
2019-05-15 08:05:44,296 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 2
2019-05-15 08:05:44,297 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 0
2019-05-15 08:05:44,303 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:44,303 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:44,607 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Sankt Gallen.
2019-05-15 08:05:44,609 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 2
2019-05-15 08:05:44,609 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 1
2019-05-15 08:05:44,624 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:45,020 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Solothurn.
2019-05-15 08:05:45,021 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 3
2019-05-15 08:05:45,022 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 0
2019-05-15 08:05:45,030 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:45,031 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:45,337 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Jura.
2019-05-15 08:05:45,343 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:45,344 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:45,662 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Genève.
2019-05-15 08:05:45,664 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:45,665 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:45,958 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Vaud.
2019-05-15 08:05:45,959 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 4
2019-05-15 08:05:45,959 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 2
2019-05-15 08:05:45,985 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:46,399 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Neuchâtel.
2019-05-15 08:05:46,404 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:46,405 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:46,716 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Bern.
2019-05-15 08:05:46,718 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 3
2019-05-15 08:05:46,718 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 1
2019-05-15 08:05:46,771 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:47,325 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Lucerne.
2019-05-15 08:05:47,334 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:47,335 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:47,687 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Zug.
2019-05-15 08:05:47,689 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:47,690 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:47,980 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Uri.
2019-05-15 08:05:47,987 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:47,988 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:48,310 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Schwyz.
2019-05-15 08:05:48,315 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:48,316 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:48,624 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Glarus.
2019-05-15 08:05:48,628 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:48,629 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:48,927 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Nidwalden.
2019-05-15 08:05:48,930 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:48,931 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:49,220 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Fribourg.
2019-05-15 08:05:49,221 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 5
2019-05-15 08:05:49,222 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 0
2019-05-15 08:05:49,234 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:49,235 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:49,592 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Obwalden.
2019-05-15 08:05:49,593 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 2
2019-05-15 08:05:49,593 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 0
2019-05-15 08:05:49,598 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:49,598 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:49,913 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Appenzell Ausserrhoden.
2019-05-15 08:05:49,916 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:49,917 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:50,212 - climada.entity.exposures.litpop - DEBUG - Caclulating admin1 for Appenzell Innerrhoden.
2019-05-15 08:05:50,213 - climada.entity.exposures.litpop - DEBUG - Detected subshapes: 3
2019-05-15 08:05:50,214 - climada.entity.exposures.litpop - DEBUG - of which detected enclaves: 0
2019-05-15 08:05:50,217 - climada.entity.exposures.litpop - DEBUG - Removing enclaves...
2019-05-15 08:05:50,218 - climada.entity.exposures.litpop - DEBUG - Successfully isolated coordinates from shape
2019-05-15 08:05:50,540 - climada.entity.exposures.litpop - INFO - Creating the LitPop exposure took 70 s
2019-05-15 08:05:50,541 - climada.entity.exposures.base - INFO - crs set to default value: {'init': 'epsg:4326', 'no_defs': True}
2019-05-15 08:05:50,542 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:05:50,543 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:05:50,544 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:05:50,544 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:05:50,545 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:05:50,546 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:05:50,585 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:05:50,586 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:05:50,586 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:05:50,587 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:05:50,588 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:05:50,589 - climada.entity.exposures.base - INFO - Setting geometry attribute.
2019-05-15 08:05:51,210 - climada.entity.exposures.base - INFO - Hazard type not set in if_
2019-05-15 08:05:51,211 - climada.entity.exposures.base - INFO - centr_ not set.
2019-05-15 08:05:51,211 - climada.entity.exposures.base - INFO - deductible not set.
2019-05-15 08:05:51,212 - climada.entity.exposures.base - INFO - cover not set.
2019-05-15 08:05:51,213 - climada.entity.exposures.base - INFO - category_id not set.
2019-05-15 08:05:51,214 - climada.entity.exposures.base - INFO - geometry not set.
2019-05-15 08:05:51,214 - climada.entity.exposures.base - INFO - Setting geometry attribute.
Done.
[12]:
# Plotting:
norm=colors.LogNorm(vmin=1.5e4, vmax=1.5e9) # setting range for the log-normal scale
ent_adm0.plot_hexbin(buffer=.3, norm=norm)
ent_adm1.plot_hexbin(buffer=.3, norm=norm)
print('admin-0: First figure')
print('admin-1: Second figure')
'''Note the small differences in eastern Switzerland.'''
/Users/aznarsig/anaconda3/envs/climada_up/lib/python3.7/site-packages/matplotlib/tight_layout.py:176: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all axes decorations.
warnings.warn('Tight layout not applied. The left and right margins '
admin-0: First figure
admin-1: Second figure
[12]:
'Note the small differences in eastern Switzerland.'
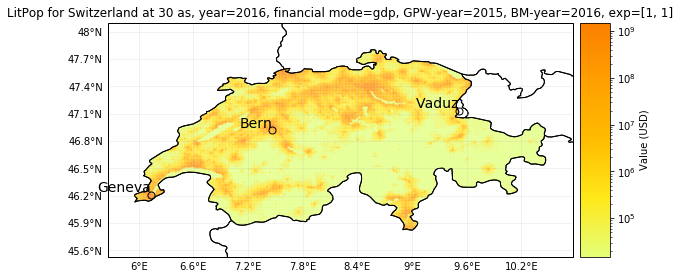
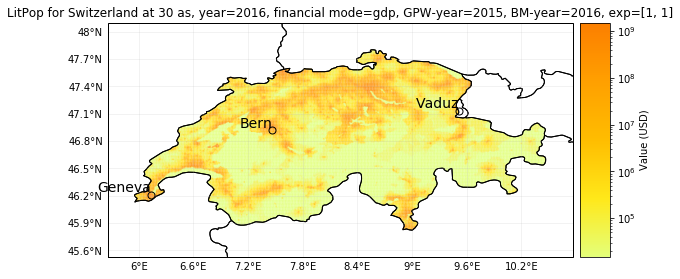
Export Exposure Data to CSV¶
The method ent.to_csv() can be used to export any CLIMADA exposure entity to a CSV-file.
Please refer to the exposure tutorial climada_entity_Exposures.ipynb for more info on the export of exposure data!
[13]:
# Export LitPop based entity to CSV:
# set output path:
import os
from climada.util.constants import DATA_DIR # default CLIMADA-data directory
output_path = os.path.join(DATA_DIR, 'export') # subfolder export
if not os.path.isdir(output_path): # create output folder if it does not exist
os.mkdir(output_path)
# set output file path:
output_path = os.path.join(output_path, 'LitPop_Exposure_Burundi_Tanzania_Rwanda.csv')
# Export data:
ent.to_csv(output_path, sep=',')
# display('Exposure entity exported to' + output_path)
# Note that the variable "region_id" in the exported CSV file is a number identifying the country, i.e.:
display('Examples for region_id:')
display('Burundi: ' + str(iso_cntry.get('BDI').numeric))
display('Tanzania: ' + str(iso_cntry.get('TZA').numeric))
display('Rwanda: ' + str(iso_cntry.get('RWA').numeric))
'Examples for region_id:'
'Burundi: 108'
'Tanzania: 834'
'Rwanda: 646'